For businesses looking to boost online sales and conversions, a countdown timer can be a powerful tool in your digital toolkit. Creating a sense of urgency is a proven psychological trick that drives people to take action. In this guide, we’ll provide a detailed overview of how you can create a countdown timer using JavaScript, outlining its advantages, potential drawbacks, and several use cases to help enhance your sales and conversions.
Table of Contents
Why a Countdown Timer?
A countdown timer is a versatile tool that can serve numerous purposes on your website:
- It can create a sense of urgency for limited-time offers, leading to quicker decisions.
- It can be used to count down to an upcoming event or product launch.
- It can give visitors a clear indication of the time left until an offer expires.
All these scenarios can encourage visitors to take action, which ultimately leads to higher conversions.
Step-by-step Guide to Creating a Countdown Timer in JavaScript
Here’s a detailed step-by-step guide on how you can create a countdown timer in JavaScript:
Setting Up the HTML Structure
First, we need to set up our HTML structure. We’ll create a simple div
element where our timer will be displayed.
<div id="countdown">
<div id="days"><span></span> days</div>
<div id="hours"><span></span> hours</div>
<div id="minutes"><span></span> minutes</div>
<div id="seconds"><span></span> seconds</div>
</div>
Creating the JavaScript Countdown Timer
Next, we’ll create the JavaScript that powers our countdown timer.
function countdown(dateEnd) {
var timer, days, hours, minutes, seconds;
dateEnd = new Date(dateEnd);
dateEnd = dateEnd.getTime();
if (isNaN(dateEnd)) {
return;
}
timer = setInterval(calculate, 1000);
function calculate() {
var dateStart = new Date();
var dateStart = new Date(dateStart.getUTCFullYear(),
dateStart.getUTCMonth(),
dateStart.getUTCDate(),
dateStart.getUTCHours(),
dateStart.getUTCMinutes(),
dateStart.getUTCSeconds());
var timeRemaining = parseInt((dateEnd - dateStart.getTime()) / 1000);
if (timeRemaining >= 0) {
days = parseInt(timeRemaining / 86400);
timeRemaining = (timeRemaining % 86400);
hours = parseInt(timeRemaining / 3600);
timeRemaining = (timeRemaining % 3600);
minutes = parseInt(timeRemaining / 60);
timeRemaining = (timeRemaining % 60);
seconds = parseInt(timeRemaining);
document.querySelector("#days > span").innerHTML = parseInt(days, 10);
document.querySelector("#hours > span").innerHTML = ("0" + hours).slice(-2);
document.querySelector("#minutes > span").innerHTML = ("0" + minutes).slice(-2);
document.querySelector("#seconds > span").innerHTML = ("0" + seconds).slice(-2);
} else {
return;
}
}
}
To use the countdown, call the countdown()
function with the end date as a parameter. The end date should be in this format: “January 30, 2024 12:00:00”.
countdown('January 30, 2024 12:00:00');
Styling
While it’s up to you to apply the styling you need or like, I include a few here just as an example.
#countdown {
display: flex;
gap: 20px;
padding: 100px;
background-color: aliceblue;
justify-content: center;
> div{
display: inline-block;
padding: 20px;
font-size: 20px;
font-weight: 500;
background: aqua;
border-radius: 10px;
}
}
And here is the result:
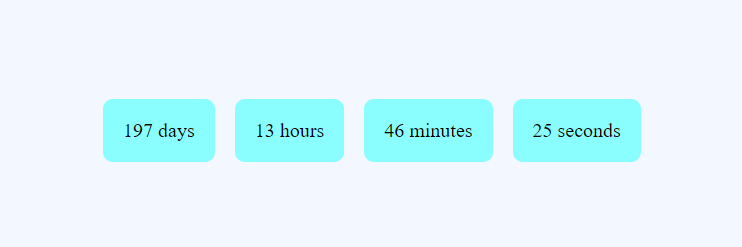
Pros and Cons of Using a Countdown Timer
Like all strategies, using a countdown timer has its benefits and potential drawbacks.
Pros:
- Creates a Sense of Urgency: Countdown timers can push customers to make faster buying decisions, reducing the chance of cart abandonment.
- Increases Engagement: Timers add an interactive element to your website, which can increase user engagement.
- Easy to Implement: With basic knowledge of JavaScript, you can easily add a countdown timer to your website.
Cons:
- Can Lead to Pressure Sales: If not used judiciously, countdown timers can seem manipulative, leading to negative customer experiences.
- Overuse Can Lead to Desensitization: If every offer on your site has a timer, customers might become desensitized, reducing the effectiveness of the strategy.
Tips to Make Your Countdown Timer More Effective
- Clear Communication: Ensure your customers know what the timer is counting down to, be it the end of a sale or the launch of a product.
- Consider Your Design: The design of your countdown timer should align with your overall website design. A misfit timer can be distracting and can lead to a decrease in user experience.
- Don’t Overuse: Countdown timers work best when used sparingly. If you have them running all the time, they can lose their effect.
Wrapping Up
Implementing a countdown timer on your website can significantly boost your sales and conversions if done correctly. By creating a sense of urgency, you motivate users to take action. Remember to use this strategy judiciously and ensure that your countdown timer aligns with your website design for the best results.
With this guide, you should now be equipped with the knowledge you need to create your countdown timer using JavaScript and boost your online sales. Happy selling!
The code snippets you provided are very succinct and easy to understand. This is great for beginners who might be intimidated by overly complex examples.